Plaid: Instant Account Verification
Step-by-step guide to integrating Dwolla’s Open Banking solution with Plaid. Learn how to enable instant account verification, streamline account-to-account (A2A) payments, and enhance security for enterprise payment workflows.
Overview
This guide dives into leveraging Dwolla’s Open Banking Services in collaboration with Plaid to streamline bank account verification within your Dwolla-powered application. Open banking empowers your users to more securely share their financial data with Dwolla and your application, eliminating the need for manual data entry and improving the overall user experience.
We’ll walk you through the steps to set up and integrate Plaid Instant Account Verification (Plaid IAV) using Dwolla’s Exchange Sessions API. Dwolla’s powerful Exchange Sessions API acts as the bridge between your application and Plaid’s Open Banking API. This established connection facilitates real-time verification of your user’s bank account details.
To gain hands-on experience, we recommend following along with the provided integration-examples sample app, which provides a practical understanding of the integration process for your own application.
Instant Account Verification (IAV)
Instant Account Verification (IAV) is a one-time process that verifies the bank account being added by an end user is open and active. At the end of this guide, you’ll obtain a Funding Source URL, which is a unique identifier that represents a bank account being used for account-to-account (A2A) payments.
Prerequisites
Before starting the integration, make sure you have completed the following prerequisites:
- Dwolla Account: Set up a Dwolla production or sandbox account.
- Create a Customer: Create a customer in your Dwolla account if you haven’t already done so. Check out the Dwolla Create a Customer API documentation for guidance.
Note: You do not need a Plaid account or contract with Plaid to leverage Plaid via Dwolla’s Open Banking Services. Dwolla handles the integration with Plaid for you, simplifying the process.
Sandbox Testing
Testing in the sandbox environment is essential before deploying Plaid Open Banking in production. The sandbox allows you to validate functionality with test data, ensuring a smooth experience for live users.
Test Credentials for Plaid Link Flow
Use the following credentials to simulate successful authentication with test banks:
- Username:
user_good
- Password:
pass_good
Returning User Testing in Sandbox
Plaid’s Sandbox includes pre-seeded test users for validating different returning user scenarios. Use the phone numbers and OTP (always 123456
) below to simulate these cases:
Scenario | Phone Number |
---|---|
New User | 415-555-0010 |
Verified Returning User | 415-555-0011 |
Verified Returning User: Linked New Account | 415-555-0012 |
Verified Returning User: Linked OAuth Bank | 415-555-0013 |
Verified Returning User + New Device | 415-555-0014 |
Verified Returning User: Auto Account Select | 415-555-0015 |
Key Notes About Plaid Test Banks
Plaid offers two test banks: one for checking accounts and one for savings accounts. Both have predefined account and routing numbers. This means:
- You can add up to two banks per (one checking, one savings).
- To test additional banks, remove an existing funding source and repeat the Plaid Link flow.
Plaid SDKs for Account Authentication
Plaid Link serves as the front-end widget for Plaid IAV, enabling users to securely authenticate and link their bank accounts. This widget can be seamlessly embedded into your application using one of Plaid’s Link client SDKs.
In this guide, we’ll be using the react-plaid-link
package, which is specifically designed for React web applications. It simplifies the integration of Plaid Link by providing a React hook to handle initialization and user interactions with the widget.
If you’re using a different framework or platform, Plaid offers SDKs tailored to various environments from which you can select the appropriate package for your application infrastructure.
Demo
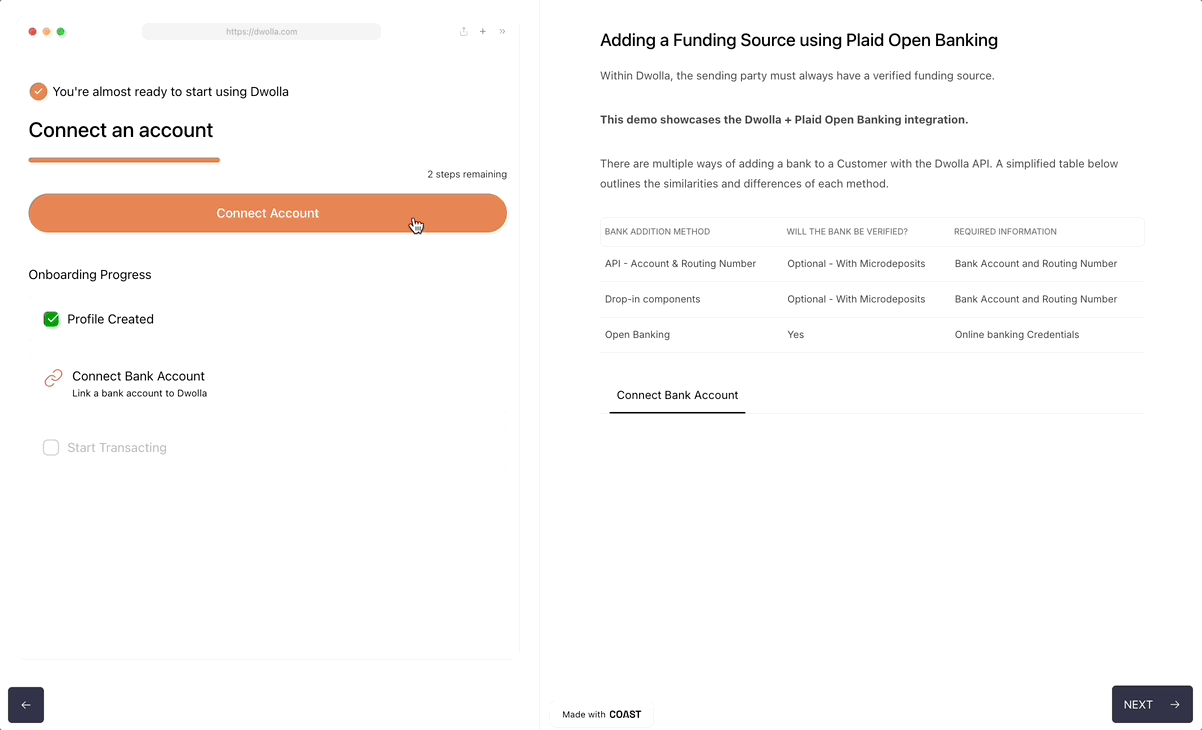
Plaid Open Banking Demo
Coast Demo: Plaid Open Banking Flow
Integration Steps
Step 1 - Initiate Exchange Session with Plaid
To begin, you will create an exchange session for your Customer in Dwolla using the Exchange Sessions API. This session will specify Plaid as the exchange partner. The Exchange Partner ID for Plaid can be found by calling the List Exchange Partners API endpoint.
About the redirect-url
Field:
If have an Android and/or iOS app, you must include a platform-specific redirect-url
in the request body when creating an exchange session. This URL determines where the user is redirected after completing the Plaid Link flow. The redirect-url
is a required field only for Android and iOS apps. It is not needed for web-based implementations.
- For Android, use the Android package name as the redirect-url value (e.g.,
com.example.app123
). - For iOS, use a valid HTTP or HTTPS URL (e.g.,
https://example.com/app123
) that can handle redirects in the app.
Dwolla will validate the provided redirect-url
based on these conventions and send the appropriate value to Plaid. If the redirect-url does not start with a valid protocol (https:// or http://), it will be assumed to be an Android package name.
Exchange Sessions are single-use. Once a user starts the IAV flow initiated by creation of a session, it becomes invalid and cannot be reused.
Example: Initiating an Exchange Session via Dwolla API
Example Request: Android
Example Request: iOS
Step 2 - Retrieve Exchange Session and Complete Plaid Link Flow
After creating the exchange session, retrieve the session details from Dwolla to obtain the Plaid Link Token. This token initializes the Plaid Link flow on the front end, where the Customer authenticates their bank account through Plaid’s secure interface. Once the flow is successfully completed, the onSuccess
handler captures the publicToken
returned by Plaid, which can be used for subsequent operations like creating an exchange.
Code Example
Backend - Backend: Retrieve Plaid Link Token
Frontend - Initialize and Handle Plaid Link Flow (using React)
Step 3 - Create Exchange with Plaid Public Token
After successfully completing the Plaid Link flow and retrieving the public token
, create an resource in the Dwolla API by passing the public token
returned by Plaid. This creates an “exchange” representing the link between the Dwolla Customer and their external bank account.
Example: Create Exchange Resource in Dwolla
Step 4 - Create Funding Source
After successfully creating the exchange, create a funding source for the Customer. This involves calling Dwolla’s Create a Funding Source endpoint, where you’ll provide the exchange resource obtained from the previous step.
In the following function, once a response is received, it will extract the Location
header value, which is the fully-qualified URL specifying the resource location of the Customers’s funding source.
Example: Create Funding Source in Dwolla
Handling Re-authentication
When Customers initially connect their bank account via Instant Account Verification, they authenticate their bank account with Plaid and grant permission to access their account information. This allows Dwolla to perform actions like checking bank balances. However, this access can be interrupted by changes made by the Customer, such as if the Customer updates their bank password, multi-factor authentication method or revokes consent to access their account information.
To maintain a smooth user experience, your application needs to handle these scenarios gracefully. Dwolla’s API provides an UpdateCredentials
error response to signal when a Customer’s bank connection needs to be refreshed. Additionally, Dwolla will also send a customer_exchange_reauth_required
webhook denoting whether an exchange is pending deactivation, or a customer_exchange_deactivated
webhook denoting that an exchange has been deactivated and requires re-authentication.
UpdateCredentials
error response
Steps to manage re-authentication
-
Detect the
UpdateCredentials
error: When making calls to Dwolla’s API (e.g., checking a bank balance), implement error handling to catch the UpdateCredentials response, which is an HTTP 400 error. -
Communicate with your Customer: It’s crucial to inform the Customer why they need to re-authenticate and how to do so. Use clear and concise language in in-app messages, emails or text messages to guide them back to your application to complete the process.
-
Initiate the re-authentication flow: Upon receiving this error, redirect the Customer to re-authenticate their bank account. This is done by creating a new re-authentication exchange session. This will guide the Customer through the necessary steps to re-establish their bank connection.
By following these steps, you can ensure that your application can handle interruptions to bank connections effectively, providing a smooth and user-friendly experience.
Initiate re-authentication exchange-session
Use Dwolla’s API endpoint to create a re-authentication exchange session, which initiates an Exchange Session for a Customer. While an optional redirect URL can be specified in the request body, it’s not required.